6.0.1
1 Type Reference
Any Racket value. All other types are subtypes of
Any.
The empty type. No values inhabit this type, and
any expression of this type will not evaluate to a value.
1.1 Base Types
1.1.1 Numeric Types
These types represent the hierarchy of numbers of Racket. The
diagram below shows the relationships between the types in the hierarchy.
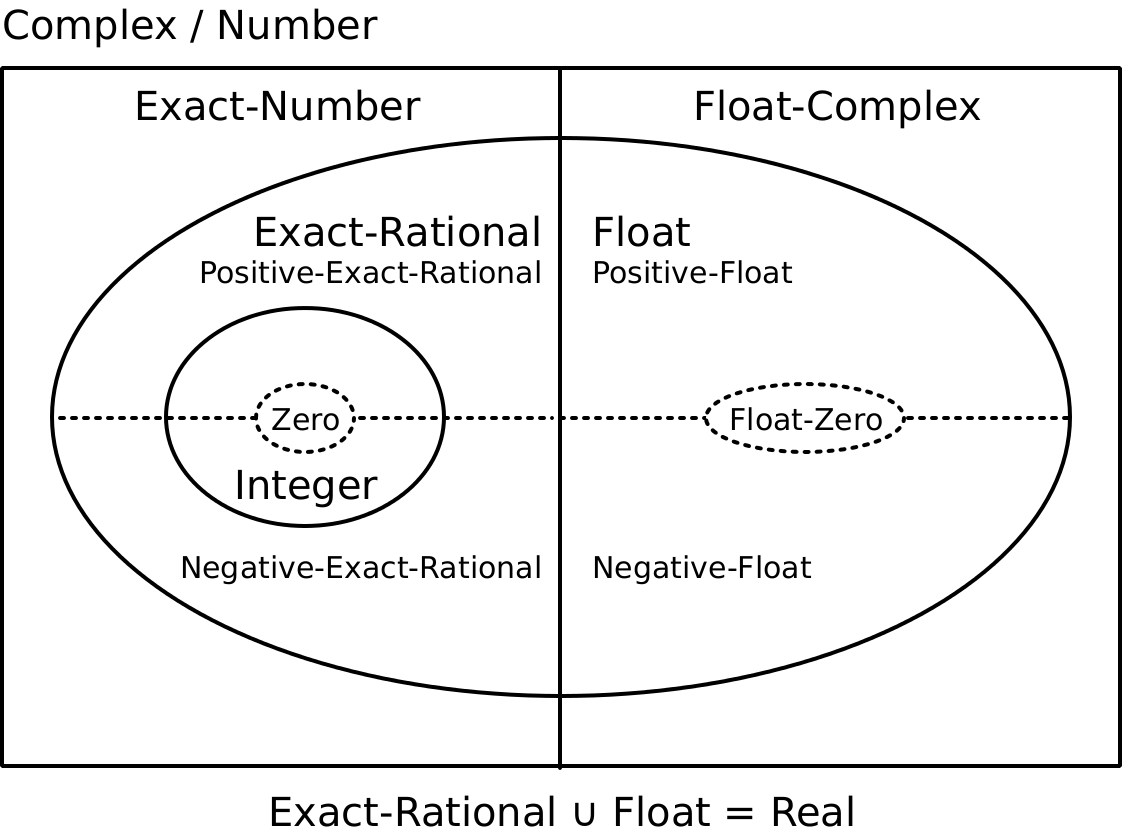
The regions with a solid border are layers of the numeric hierarchy
corresponding to sets of numbers such as integers or rationals. Layers
contained within another are subtypes of the layer containing them. For
example, Exact-Rational is a subtype of Exact-Number.
The Real layer is also divided into positive and negative types
(shown with a dotted line). The Integer layer is subdivided into
several fixed-width integers types, detailed later in this section.
Number and
Complex are synonyms. This is the most general
numeric type, including all Racket numbers, both exact and inexact, including
complex numbers.
Includes Racket’s exact integers and corresponds to the
exact-integer? predicate. This is the most general type that is still
valid for indexing and other operations that require integral values.
Includes Racket’s double-precision (default) floating-point numbers and
corresponds to the
flonum? predicate. This type excludes
single-precision floating-point numbers.
Includes Racket’s single-precision floating-point numbers and corresponds to
the
single-flonum? predicate. This type excludes double-precision
floating-point numbers.
Includes all of Racket’s floating-point numbers, both single- and
double-precision.
Includes Racket’s exact rationals, which include fractions and exact integers.
Includes all of Racket’s real numbers, which include both exact rationals and
all floating-point numbers. This is the most general type for which comparisons
(e.g.
<) are defined.
These types correspond to Racket’s complex numbers.
The above types can be subdivided into more precise types if you want to
enforce tighter constraints. Typed Racket provides types for the positive,
negative, non-negative and non-positive subsets of the above types (where
applicable).
These types are useful when enforcing that values have a specific
sign. However, programs using them may require additional dynamic checks when
the type-checker cannot guarantee that the sign constraints will be respected.
In addition to being divided by sign, integers are further subdivided into
range-bounded types. The relationships between most of the range-bounded types
are shown in this diagram:
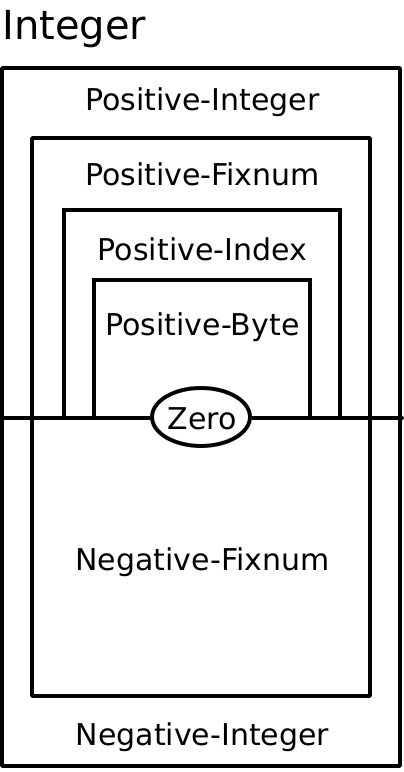
Like the previous diagram, types nested inside of another in the
diagram are subtypes of its containing types.
One includes only the integer
1.
Byte includes
numbers from
0 to
255.
Index is bounded by
0 and by the length of the longest possible Racket
vector.
Fixnum includes all numbers represented by Racket as machine
integers. For the latter two families, the sets of values included in the types
are architecture-dependent, but typechecking is architecture-independent.
These types are useful to enforce bounds on numeric values, but given the
limited amount of closure properties these types offer, dynamic checks may be
needed to check the desired bounds at runtime.
Examples: |
> 7 | - : Integer [more precisely: Positive-Byte] | 7 | > 8.3 | - : Flonum [more precisely: Positive-Flonum] | 8.3 | > (/ 8 3) | - : Exact-Rational [more precisely: Positive-Exact-Rational] | 8/3 | > 0 | - : Integer [more precisely: Zero] | 0 | > -12 | - : Integer [more precisely: Negative-Fixnum] | -12 | > 3+4i | - : Exact-Number | 3+4i |
|
1.1.2 Other Base Types
These types represent primitive Racket data.
Examples: |
> #t | - : Boolean [more precisely: True] | #t | > #f | - : False | #f | > "hello" | - : String | "hello" | > (current-input-port) | - : Input-Port | #<input-port:string> | > (current-output-port) | - : Output-Port | #<output-port:string> | > (string->path "/") | - : Path | #<path:/> | > #rx"a*b*" | - : Regexp | #rx"a*b*" | > #px"a*b*" | - : PRegexp | #px"a*b*" | > '#"bytes" | - : Bytes | #"bytes" | > (current-namespace) | - : Namespace | #<namespace:0> | > #\b | - : Char | #\b | > (thread (lambda () (add1 7))) | - : Thread | #<thread> |
|
The union of the
Path and
String types. Note that this does not
match exactly what the predicate
path-string?
recognizes. For example, strings
that contain the character
#\nul have the type
Path-String but
path-string? returns
#f for those strings. For a complete specification
of which strings
path-string? accepts, see its
documentation.
1.2 Singleton Types
Some kinds of data are given singleton types by default. In
particular, booleans, symbols, and keywords have types which
consist only of the particular boolean, symbol, or keyword. These types are
subtypes of Boolean, Symbol and Keyword, respectively.
Examples: |
> #t | - : Boolean [more precisely: True] | #t | > '#:foo | - : '#:foo | '#:foo | > 'bar | - : Symbol [more precisely: 'bar] | 'bar |
|
1.3 Containers
The following base types are parametric in their type arguments.
is the
pair containing
s as the
car
and
t as the
cdrExamples: |
> (cons 1 2) | - : (Pairof One Positive-Byte) | '(1 . 2) | > (cons 1 "one") | - : (Pairof One String) | '(1 . "one") |
|
is the type of the list with one element, in order,
for each type provided to the
List type constructor.
(List t ... trest ... bound)
|
is the type of a list with
one element for each of the
ts, plus a sequence of elements
corresponding to
trest, where
bound
must be an identifier denoting a type variable bound with
....
Examples: |
> (list 'a 'b 'c) | - : (Listof (U 'a 'b 'c)) [more precisely: (List 'a 'b 'c)] | '(a b c) | | - : (All (a ...) | (-> Symbol (Boxof a) ... a (Pairof Symbol (List (Boxof a) ... a)))) |
| #<procedure> | > (map symbol->string (list 'a 'b 'c)) | - : (Listof String) [more precisely: (Pairof String (Listof String))] | '("a" "b" "c") |
|
is the type of a
mutable pair with unknown
element types and is the supertype of all mutable pair types.
This type typically appears in programs via the combination of
occurrence typing and
mpair?.
Example: |
> (box "hello world") | - : (Boxof String) | '#&"hello world" |
|
is the type of a
box with an unknown element
type and is the supertype of all box types. Only read-only box operations
(e.g.
unbox) are allowed on values of this type. This type
typically appears in programs via the combination of occurrence
typing and
box?.
is the type of the list with one element, in order,
for each type provided to the
Vector type constructor.
Examples: |
> (vector 1 2 3) | - : (Vector Integer Integer Integer) | '#(1 2 3) | > #(a b c) | - : (Vector Symbol Symbol Symbol) | '#(a b c) |
|
Example: |
> (flvector 1.0 2.0 3.0) | - : FlVector | (flvector 1.0 2.0 3.0) |
|
Example: |
> (fxvector 1 2 3) | - : FxVector | (fxvector 1 2 3) |
|
is the type of a
vector with unknown length and
element types and is the supertype of all vector types.
Only read-only vector operations (e.g.
vector-ref)
are allowed on values of this type. This type typically appears in programs
via the combination of occurrence typing and
vector?.
is the type of a
hash table with key type
k and value type
v.
Example: |
> #hash((a . 1) (b . 2)) | - : (HashTable Symbol Integer) | '#hash((a . 1) (b . 2)) |
|
is the type of a
hash table with unknown key
and value types and is the supertype of all hash table types. Only read-only
hash table operations (e.g.
hash-ref) are allowed on values of this type. This type typically
appears in programs via the combination of occurrence typing and
hash?.
is the type of a
set of
t.
Example: |
> (set 0 1 2 3) | - : (Setof Byte) | (set 0 1 2 3) |
|
A
channel on which only
ts can be sent.
is the type of a
channel with unknown
message type and is the supertype of all channel types. This type typically
appears in programs via the combination of occurrence typing and
channel?.
A
parameter of
t. If two type arguments are supplied,
the first is the type the parameter accepts, and the second is the type returned.
Example: |
> (delay 3) | - : (Promise Positive-Byte) | #<promise:eval:43:0> |
|
A
future which produce a value of type
t when touched.
A
sequence that produces values of
type
t on each iteration.
is the type of a
thread cell with unknown
element type and is the supertype of all thread cell types. This type typically
appears in programs via the combination of occurrence typing and
thread-cell?.
1.4 Syntax Objects
The following types represent syntax objects and their content.
A syntax object with content of type
t.
Applying
syntax-e to a value of type
(Syntaxof t) produces a
value of type
t.
1.5 Control
The following types represent prompt tags and
keys for continuation marks for use with delimited continuation
functions and continuation mark functions.
A prompt tag to be used in a continuation prompt whose body
produces the type s and whose handler has the type
t. The type t must be a function type.
The domain of t determines the type of the values
that can be aborted, using abort-current-continuation,
to a prompt with this prompt tag.
is the type of a
prompt tag with unknown
body and handler types and is the supertype of all prompt tag types. This type
typically appears in programs via the combination of occurrence typing
and
continuation-prompt-tag?.
is the type of a continuation mark
key with unknown element type and is the supertype of all continuation mark key
types. This type typically appears in programs
via the combination of occurrence typing and
continuation-mark-key?.
1.6 Other Type Constructors
(-> dom ... rng)
|
(-> dom ... rest * rng) |
(-> dom ... rest ooo bound rng) |
(-> dom rng : pred) |
(dom ... -> rng) |
(dom ... rest * -> rng) |
(dom ... rest ooo bound -> rng) |
(dom -> rng : pred) |
|
ooo | | = | | ... | | | | | | dom | | = | | type | | | | | | mandatory-kw | | | | | | optional-kw | | | | | | mandatory-kw | | = | | keyword type | | | | | | optional-kw | | = | | [keyword type] |
|
The type of functions from the (possibly-empty)
sequence dom .... to the rng type.
Examples: |
> (λ: ([x : Number]) x) | - : (-> Number Number) | #<procedure> | > (λ: () 'hello) | - : (-> 'hello) | #<procedure> |
|
The second form specifies a uniform rest argument of type rest, and the
third form specifies a non-uniform rest argument of type
rest with bound bound. The bound refers to the type variable
that is in scope within the rest argument type.
Examples: |
> (λ: ([x : Number] y : String *) (length y)) | - : (-> Number String * Index) | #<procedure> | > ormap | - : (All (a c b ...) | (-> (-> a b ... b c) (Listof a) (Listof b) ... b (U False c))) |
| #<procedure:ormap> |
|
In the third form, the ... introduced by ooo is literal,
and bound must be an identifier denoting a type variable.
In the fourth form, there must be only one dom and pred is the type
checked by the predicate. The type pred is known as a filter for
the function type (for an introduction to filters, see
Filters and Predicates).
Example: |
> string? | - : (-> Any Boolean : String) | #<procedure:string?> |
|
The doms can include both mandatory and optional keyword arguments.
Mandatory keyword arguments are a pair of keyword and type, while optional
arguments are surrounded by a pair of parentheses.
The type of functions can also be specified with an infix ->
which comes immediately before the rng type. The fifth through
eighth forms match the first four cases, but with the infix style of arrow.
(->* (mandatory-dom ...) optional-doms rest rng)
|
|
mandatory-dom | | = | | type | | | | | | keyword type | | | | | | optional-doms | | = | | | | | | | | (optional-dom ...) | | | | | | optional-dom | | = | | type | | | | | | keyword type | | | | | | rest | | = | | | | | | | | #:rest type |
|
Constructs the type of functions with optional or rest arguments. The first
list of mandatory-doms correspond to mandatory argument types. The list
optional-doms, if provided, specifies the optional argument types.
If provided, the rest expression specifies the type of
elements in the rest argument list.
Both the mandatory and optional argument lists may contain keywords paired
with types.
The syntax for this type constructor matches the syntax of the ->*
contract combinator, but with types instead of contracts.
These are filters that can be used with
->.
Top is the filter with no information.
Bot is the filter which means the result cannot happen.
is the supertype of all function types. The
Procedure
type corresponds to values that satisfy the
procedure? predicate.
Because this type encodes
only the fact that the value is a procedure, and
not its argument types or even arity, the type-checker cannot allow values
of this type to be applied.
For the types of functions with known arity and argument types, see the ->
type constructor.
Examples: |
> (: my-list Procedure) | | > (define my-list list) | | > (my-list "zwiebelkuchen" "socca") | eval:73:0: Type Checker: Cannot apply expression of type | Procedure, since it is not a function type | in: "socca" |
|
is the union of the types
t ....
Example: |
> (λ: ([x : Real])(if (> 0 x) "yes" 'no)) | - : (-> Real (U String 'no)) | #<procedure> |
|
is a function that behaves like all of
the
fun-tys, considered in order from first to last. The
fun-tys must all be function
types constructed with
->.
is the instantiation of the parametric type
t at types
t1 t2 ...
(All (a ...) t)
|
(All (a ... a ooo) t) |
is a parameterization of type
t, with
type variables
v .... If
t is a function type
constructed with infix
->, the outer pair of parentheses
around the function type may be omitted.
Examples: |
> (: list-length : (All (A) (Listof A) -> Natural)) | | | | > (list-length (list 1 2 3)) | - : Integer [more precisely: Nonnegative-Integer] | 3 |
|
is the type of a sequence of multiple values, with
types
t .... This can only appear as the return type of a
function.
Example: |
> (values 1 2 3) | - : (values Integer Integer Integer) [more precisely: (Values One Positive-Byte Positive-Byte)] | |
|
where v is a number, boolean or string, is the singleton type containing only that value
where val is a Racket value, is the singleton type containing only that value
where i is an identifier can be a reference to a type
name or a type variable
is a recursive type where n is bound to the
recursive type in the body t
is a type which is a supertype of all instances of the
potentially-polymorphic structure type
st. Note that structure
accessors for
st will
not accept
(Struct st) as an
argument.
is a type for the structure type descriptor value
for the structure type
st. Values of this type are used with
reflective operations such as
struct-type-info.
Examples: |
> struct:arity-at-least | - : (StructType arity-at-least) | #<struct-type:arity-at-least> | > (struct-type-info struct:arity-at-least) | - : (values | Symbol | Integer | Integer | (-> arity-at-least Nonnegative-Integer Any) | (-> arity-at-least Nonnegative-Integer Nothing Void) | (Listof Nonnegative-Integer) | (U False Struct-TypeTop) | Boolean) | [more precisely: (values | Symbol | Nonnegative-Integer | Nonnegative-Integer | (-> arity-at-least Nonnegative-Integer Any) | (-> arity-at-least Nonnegative-Integer Nothing Void) | (Listof Nonnegative-Integer) | (U False Struct-TypeTop) | Boolean)] |
| 'arity-at-least | 1 | 0 | #<procedure:arity-at-least-ref> | #<procedure:arity-at-least-set!> | '(0) | #f | #f |
|
|
is the supertype of all types for structure type
descriptor values. The corresponding structure type is unknown for values of
this top type.
1.7 Other Types
Either t or #f